Get ready to explore PHP and enhance your web development skills. Whether you’re a seasoned developer seeking a refresher or a newcomer eager to dive into the world of web programming, this guide is here to help. Covering everything from the basics to advanced topics, you’ll be equipped to handle projects of any size – from small-scale ventures to large enterprise applications. PHP’s versatility makes it a dynamic language that continues to play a significant role in shaping the digital landscape. Join us on this journey through PHP, from A to Z!
What is PHP?
Why has PHP remained a popular choice in web development?
PHP remains a popular choice in web development despite new languages and frameworks. Let’s explore why developers worldwide still prefer PHP for building dynamic and interactive web applications:
- Ease of Learning and Use: PHP is popular because it’s easy for beginners. Its simple syntax makes it quick for developers to start building web applications without the steep learning curve found in other languages.
- Embedding in HTML: PHP’s integration into HTML is a game-changer in web development. It lets developers mix PHP code with HTML, making it easy to create dynamic web pages. This seamless integration reduces the need for separating code extensively, making development more efficient.
- Community and Resources: The PHP community is crucial for its success, with many active users providing abundant resources like documentation, tutorials, forums, and open-source projects. This collaborative environment helps developers find solutions to challenges easily and stay informed about best practices.
- Frameworks: PHP has improved with the addition of strong frameworks like Laravel, Symfony, and CodeIgniter. These frameworks help developers build applications more efficiently by providing structure, reusable parts, and following best practices, saving time and effort on projects of different sizes.
- Cross-Platform Compatibility and Integration: PHP works well with databases like MySQL and PostgreSQL, making it versatile for web development. It also easily integrates with other technologies, allowing developers to build full-stack applications and enhancing PHP’s flexibility and adaptability. It is compatible with various operating systems, including Windows, Linux, macOS, and others.
- Cost-Effectiveness: PHP is free to use, making it cost-effective for businesses, especially smaller ones and startups with budget constraints. The absence of licensing costs has contributed to PHP’s popularity in various development projects.
- Performance Improvements: PHP 7, with improvements like Zend Engine 3 and better memory usage, significantly boosted performance. This made PHP more competitive in terms of execution speed compared to other languages.
- Host Provider Support: PHP is widely supported by hosting providers, making it easy for developers to deploy and host PHP applications. This widespread availability provides developers with many hosting choices, contributing to the widespread use of PHP online.
- Legacy Systems and Maintenance: Many websites and apps were made with PHP, creating a big pile of old code. Companies prefer sticking with PHP to update and maintain these systems, avoiding the complications and expenses of switching to a new technology.
- Versatility: PHP stays relevant because it’s versatile, fitting into different web projects – be it basic websites or complex applications. Its flexibility and compatibility with diverse project needs make it a handy tool for developers.
A: Array Adventures
In PHP, an array is a versatile and fundamental data structure that allows you to store and manage multiple values under a single variable name. PHP arrays can hold various types of data, such as numbers, strings, or even other arrays. Here’s a brief overview of working with arrays in PHP:
Creating an Array:
Creating an array in PHP involves defining a variable that can hold multiple values. There are two primary methods for creating arrays in PHP: using the array() construct or using the shorthand [] syntax.
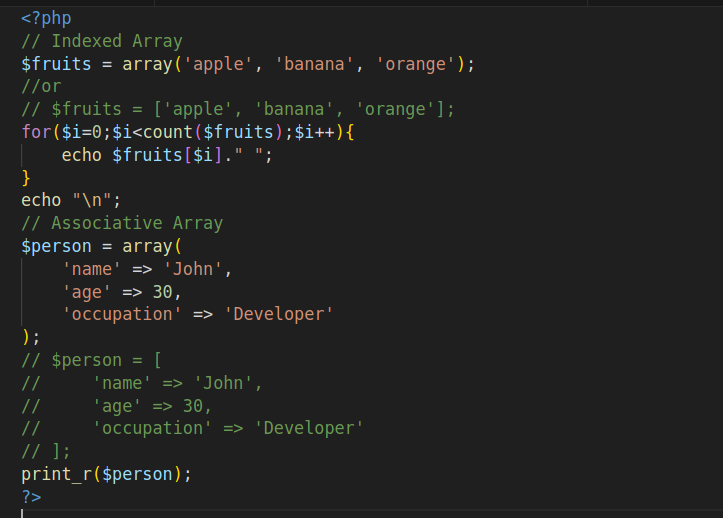
Accessing Array Elements:
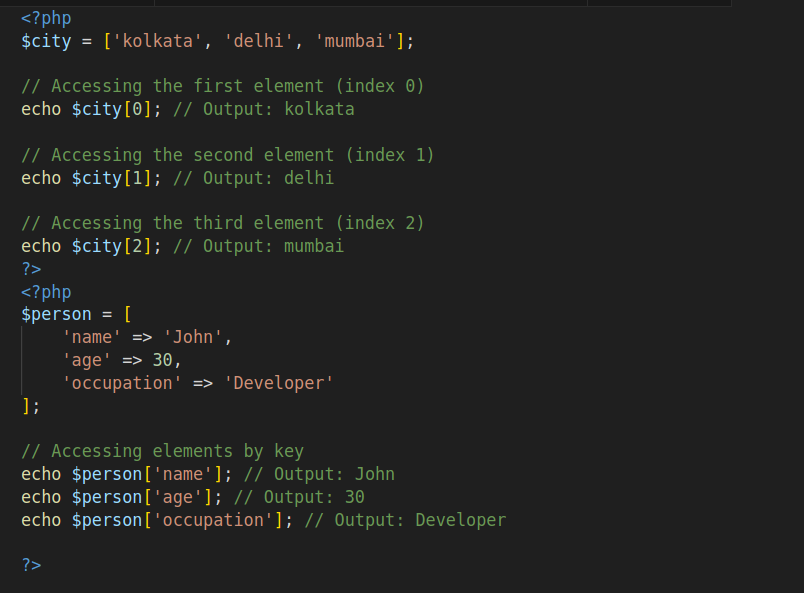
Adding Elements to an Array:
- In PHP, We can add elements to the end of an array using square bracket syntax ([]).
- The `array_push()` function is used to append one or more elements to the end of an array.
- We can also add elements at a specific index in the array using square bracket syntax.

Removing Elements from an Array :
The `unset()` function in PHP is like a delete button for variables. When you use it on an array element, it erases that specific part from the array.
The `array_splice()` function in PHP is flexible because it can remove multiple elements from an array and even add new elements in their place if required.
If we want to take out the very last item from a list of things (array), we can use the `array_pop()` function.
- Similar to array_pop(), array_shift() removes the first element from an array. `array_shift()` removes and returns the first element of the array.
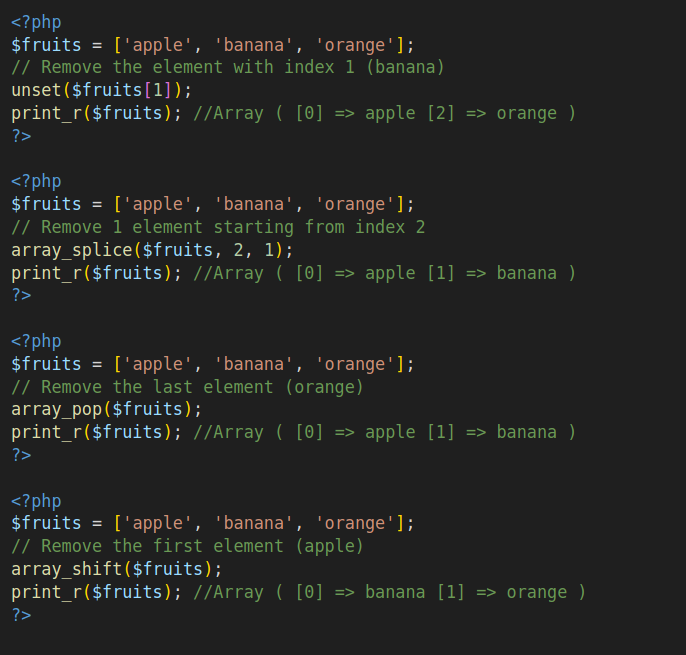
Iterating Over an Array :
// code to be executed for each $value
}
““
- $array: The array you want to iterate over.
- $value: A variable representing the current element’s value in each iteration.
We need both the keys and values for the Associative array during iteration.
““
foreach ($array as $key => $value) {
// code to be executed for each $key and $value
}
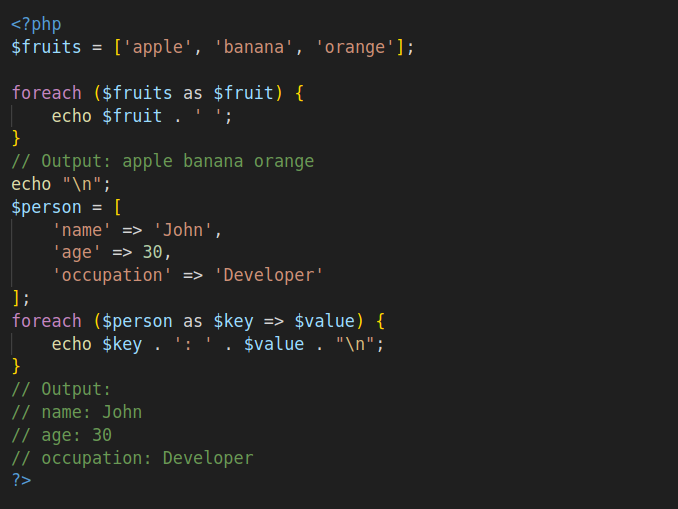
Associative Arrays:
In PHP, an associative array is like a list where each item has its own name (key) instead of a number. This makes it handy for keeping things organized, and we can easily find and use the items by their names.
We can create an associative array using the array() construct or the shorthand [] syntax. Each element in the array has a key and a corresponding value separated by the => (double arrow) symbol.
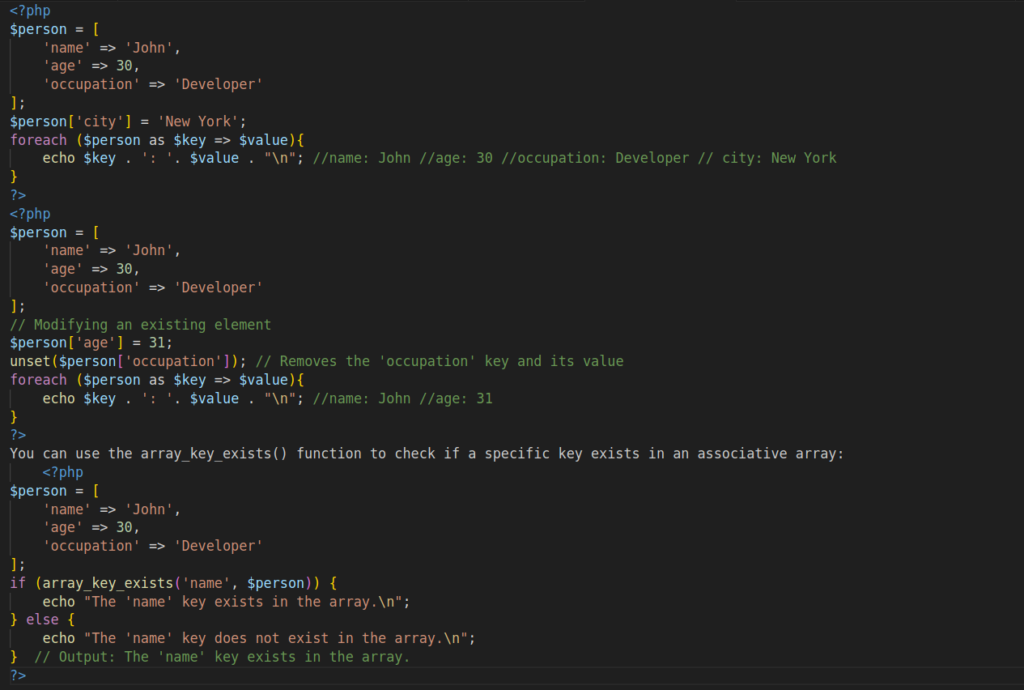
Multidimensional Arrays :
Multidimensional arrays in PHP are like containers within containers. Instead of just having a list of things in one row, we can have rows of lists. It’s a way to organize information in a more structured manner, and we use multiple numbers to find exactly what we need within these organized lists.
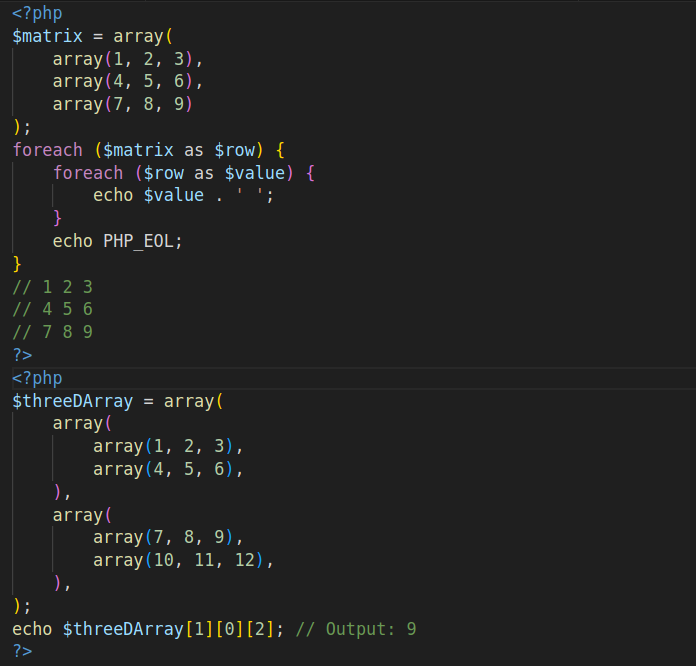
Array Functions :
PHP provides many built-in functions for array manipulation, such as count(), sort(), array_merge(), etc.
- count($array): Returns the number of elements in an array.
- array_merge($array1, $array2, …): Combines two or more arrays into a single array.
- sort($array): Sorts an array in ascending order.
- array_reverse($array): Reverses the order of elements in an array.
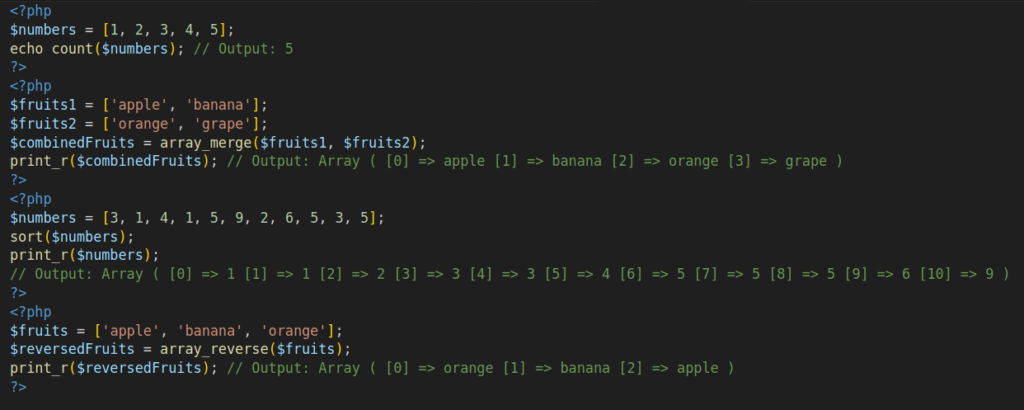
B: Building Blocks of PHP
Building blocks in programming are like the basic tools we need to build something. In simple terms, they are like the basic pieces or ideas we need to put together when creating something with code. When working with PHP, these building blocks are the essential concepts we should know, such as variables (for storing data), data types (like numbers or text), constants (values that don’t change), operators (for doing actions like adding or comparing), control structures (ways to control the flow of your program), functions (reusable blocks of code), arrays (for storing lists of items), strings (text), classes, and objects (for organizing and structuring your code), file handling functions (for working with files), error handling mechanisms (for dealing with mistakes), and superglobals (special variables that hold information about your program). Learning and understanding these building blocks is important for anyone using PHP, as we form the foundation for programming in this language.
Let’s discuss about the key points of building blocks of PHP:
Variables
- In PHP, a variable name always begins with a dollar sign (`$`), followed by the name you choose.
- In PHP, variable names are case-sensitive. This means that `$name` and `$Name` are considered as two different variables.
- Variable names in PHP can have letters, numbers, and underscores. However, we must begin with a letter or an underscore.
- When naming variables in PHP, use camelCase or snake_case for multi-word names, and make sure the names are meaningful and reflect the purpose of the variable.
- In PHP, we can directly include variables in double-quoted strings, and we can also concatenate strings using the dot (`.`) operator.
- In PHP, a variable can either be local or global. When we declare a variable inside a function, it is only accessible within that function by default. However, if we want to use the variable outside the function, we need to declare it as `global` within the function.
- In PHP, we don’t have to tell the computer what type of information our variable will hold beforehand. The variable figures it out on its own based on what we put in it.
- In PHP, variable variables allow us to create variables with dynamic names. This means we can use the value of a variable as the name for another variable. Variable variables are denoted by the double dollar sign $$.
- Unset: In PHP, using `unset()` makes a variable no longer exist. It’s like saying, “Forget about this variable; it’s gone.”
- Null: In PHP, a variable of data type `null` explicitly means it doesn’t have a value. It’s like saying, “This variable exists, but it represents nothing at the moment.”
- In PHP, a static variable is a special kind of variable tied to a function or method, not to an instance of a class. It remembers its value between different times the function or method is used. To declare a static variable, we use the keyword ‘static’ inside the function or method where it’s needed.
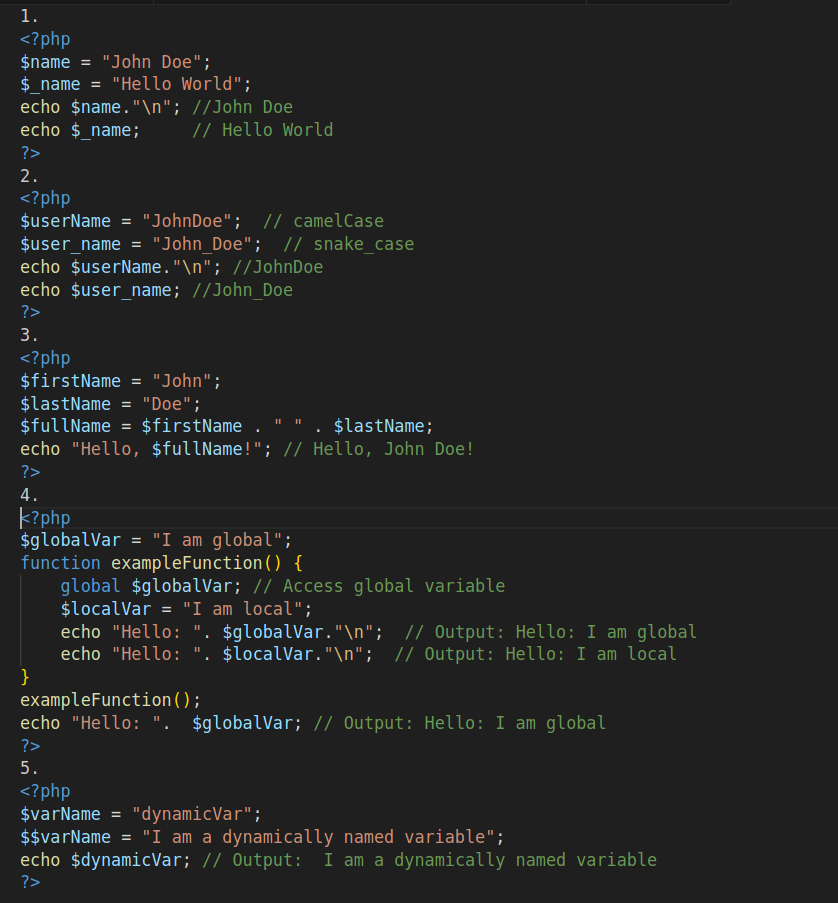
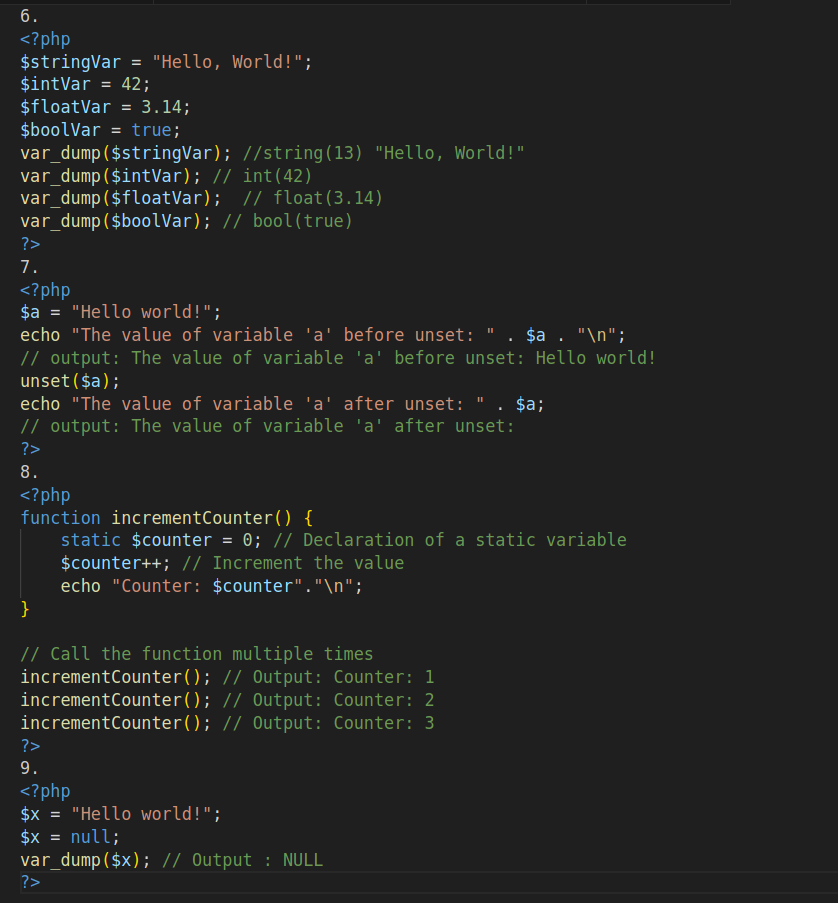
Data Types
PHP supports several data types, which are used to represent different kinds of values. Here are the main data types in PHP:
- Integer: Represents whole numbers without decimal points. ( $age = 25 ; )
- Float (Floating-point numbers or Doubles): Represents numbers with decimal points. ( $price = 3.14; )
- String: Represents sequences of characters. ( $name = “John”; )
- Boolean: Represents either true or false. ( $isAdult = true; )
- Array: Represents an ordered map that holds values. ( $colors: array(“red”, “green”, “blue”); )
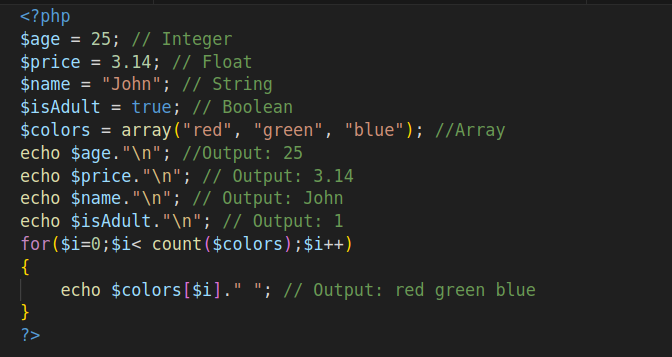
6. Object: Represents instances of user-defined classes.
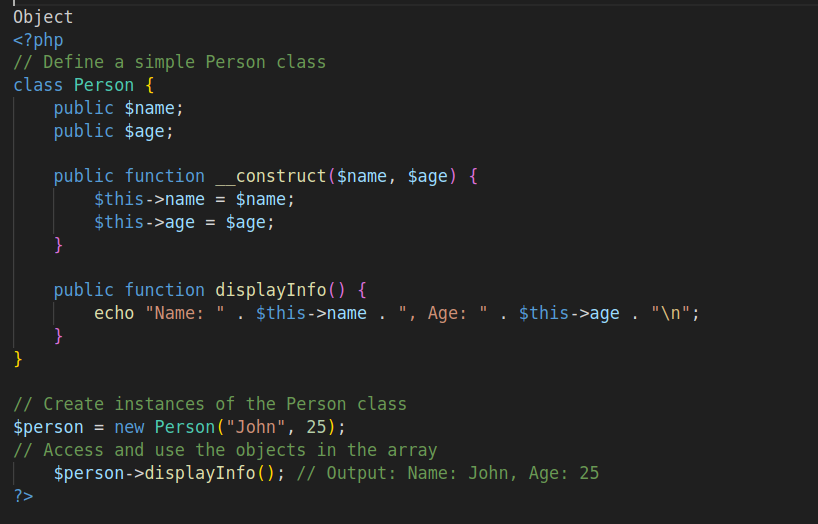
7. Resource: In PHP, the resource data type is a special type used to hold a reference to an external resource, such as a database connection, file handle, or network socket. ($file = fopen(“example.txt”, “r”); )
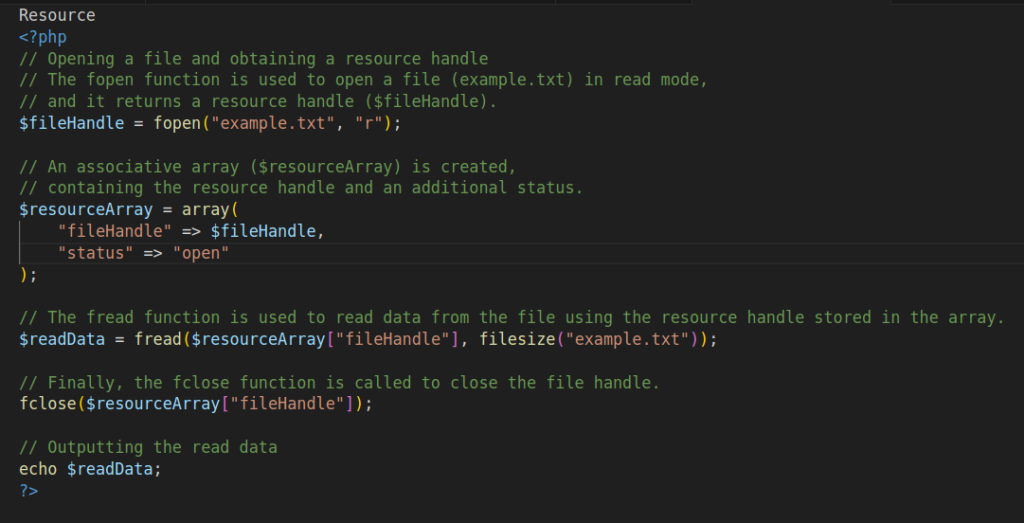
8. Null: Represents the absence of a value. ( $variable = null; )
9. Callable: Represents a callable entity, such as a function or a method. ( $func = function() { echo “Hello!”; }; )
10. Iterable: Represents an object that can be iterated (e.g., arrays or objects implementing the Traversable interface)
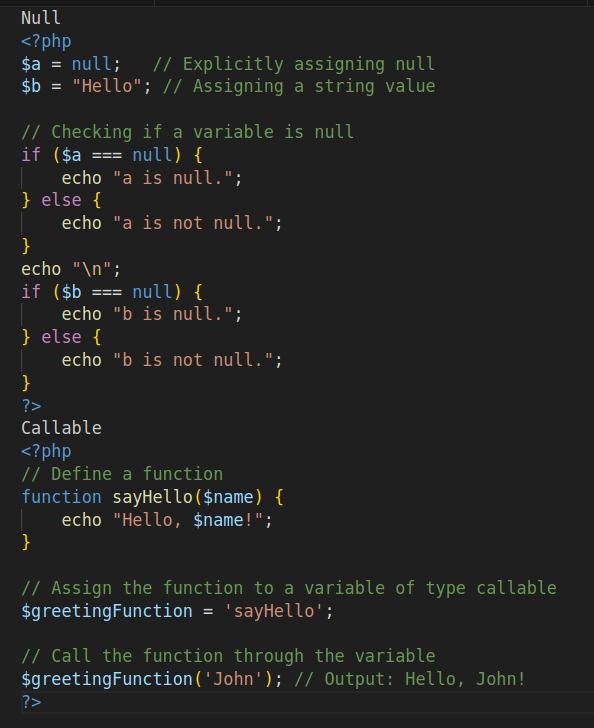
Constants
In PHP, a constant is a name or an identifier for a simple value. Once a constant is defined, its value cannot be changed during the execution of the script. Constants are case-sensitive by default, and by convention, we often write in uppercase & use it for values that remain constant throughout the execution of a script, such as configuration settings, mathematical constants, or any other value that should not be modified during the script’s runtime.
define(“CONSTANT_NAME”, “constant_value”);
- CONSTANT_NAME: The name of the constant, typically written in uppercase.
- constant_value: The value assigned to the constant.
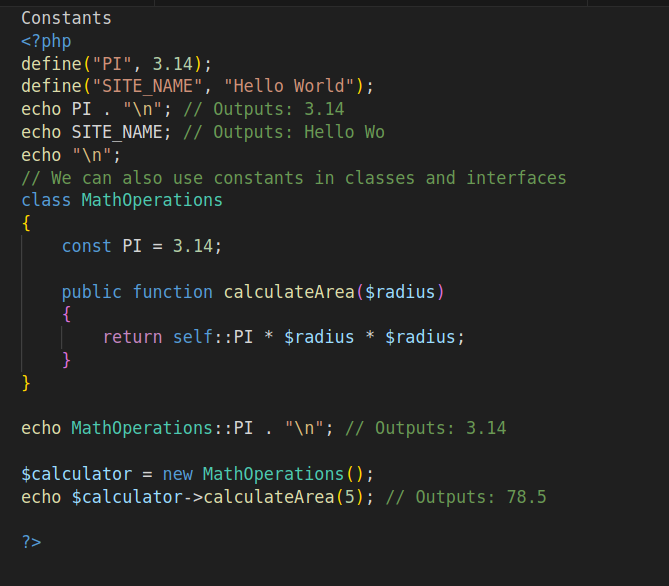
Operators
In PHP, operators are symbols or keywords that perform operations on variables and values. We are used to manipulate data, perform calculations, and make decisions in our code.
- Arithmetic Operators: Used for basic mathematical operations.
- Assignment Operators: Used to assign values to variables.
- Comparison Operators: Used to compare two values.
- Logical Operators: Used to perform logical operations.
- Increment/Decrement Operators: Used to increase or decrease the value of a variable.
- String Operators: Used for concatenating strings.
- Array Operators: Used for manipulating arrays.
- Ternary Operator: A shorthand way of writing an if-else statement.
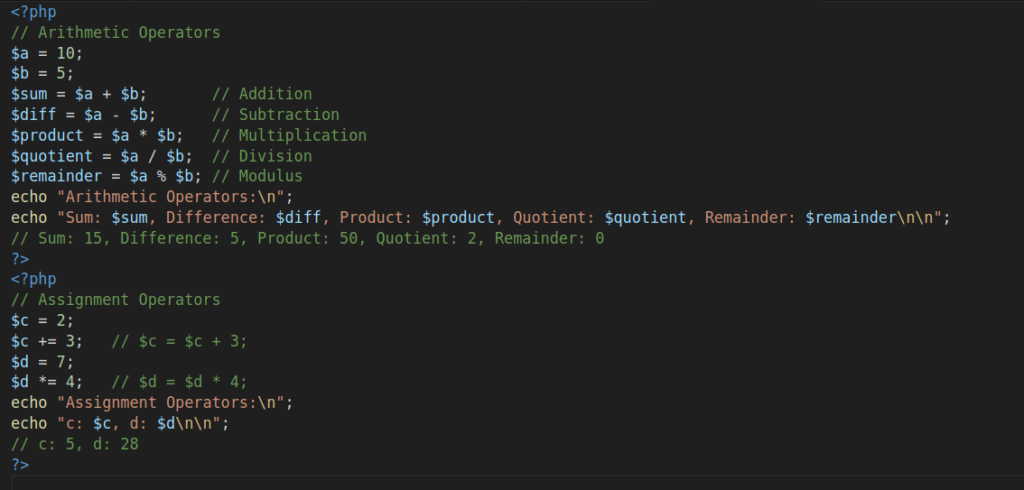
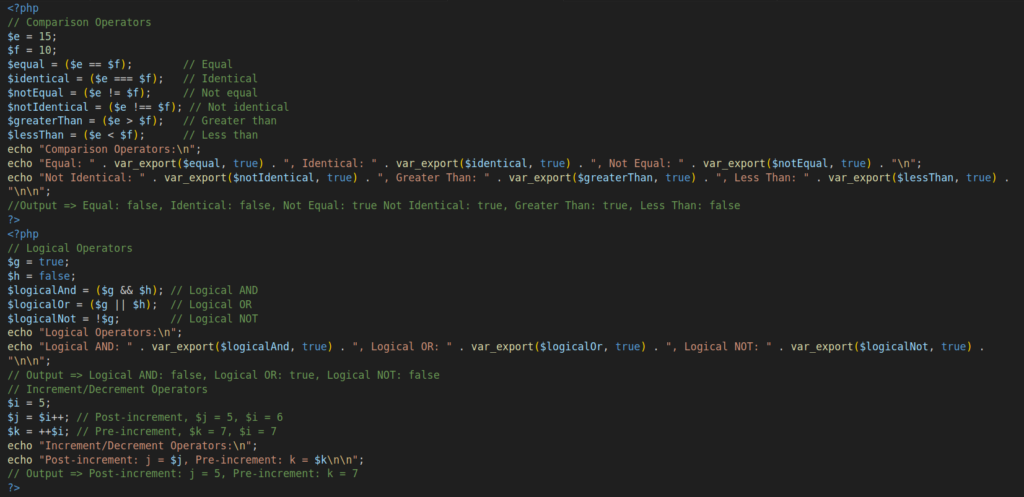
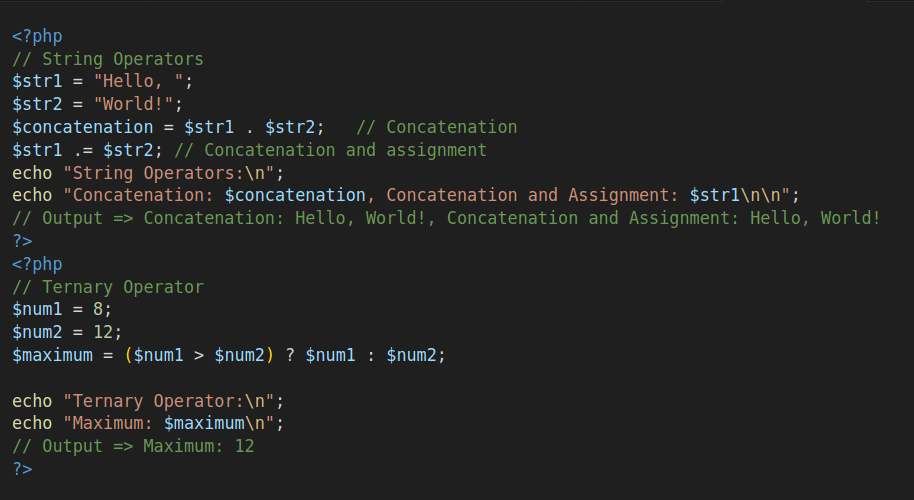
Control structures
Control structures in PHP help us manage how our code runs. They let us make decisions using structures like “if”, “else”, and “switch.” We can also repeat actions using loops like “while”, “for”, and “foreach.” These structures give us control over how our program flows based on conditions and repetitions.
- if-else: Allows us to execute different blocks of code based on a specified condition.
- elseif: Used in conjunction with if to add multiple conditions.
- switch: Allows us to test a variable against different values and execute different code blocks based on the matched case.
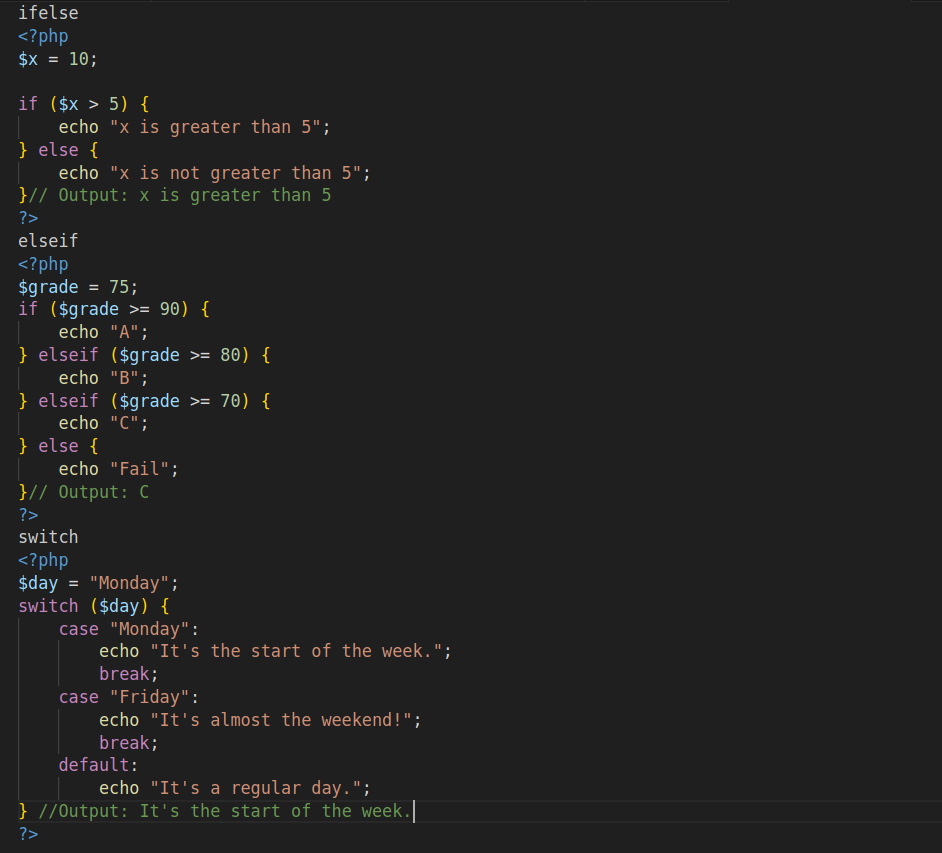
- while: Executes a block of code as long as the specified condition is true.
- do-while: Similar to while, but the condition is checked after the execution of the block, so it always executes at least once.
- for: Executes a block of code for a specified number of times.
- foreach: Used to iterate over arrays and objects.
- break and continue: break is used to exit a loop prematurely and continue is used to skip the rest of the code inside a loop for the current iteration.
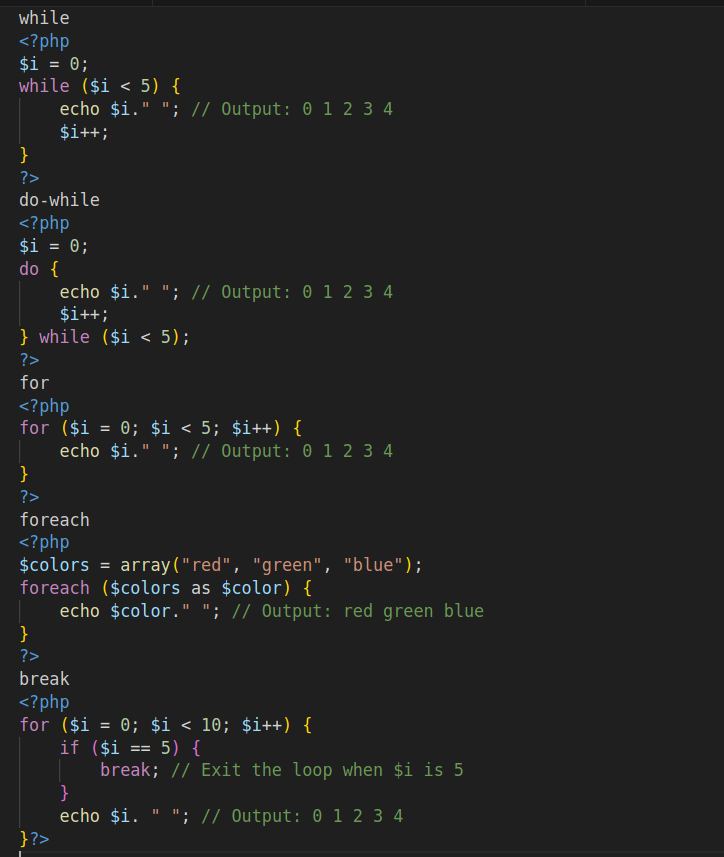
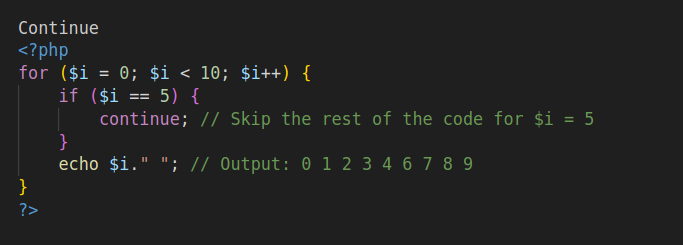
Functions
Functions in PHP are like tools that you create once and then use whenever you need to perform a specific task in your code. They make your code neat, and easy to understand and allow you to use the same set of instructions many times, making your code easier to manage.
Function Declaration
““
function functionName($parameter1, $parameter2) {
// code to be executed
return $result; // optional
}
““
- Function Name: In PHP, function names follow the same rules as variable names.
- Parameters: In PHP, parameters are the values that a function accepts when it is called. They allow us to pass information into a function, enabling the function to perform operations on that data. PHP functions can have zero or more parameters, and we declare them within the parentheses following the function name.
- Function Body: The function body in PHP is the block of code enclosed within curly braces {} that defines the behavior of the function when it is called. It contains the statements and instructions that are executed when the function is invoked.
- Return Statement: In PHP, the return statement is used in functions to explicitly specify a value that the function should return. It allows a function to pass data back to the code that called it. The return statement also terminates the execution of the function, so any code following the return statement within the function will not be executed.
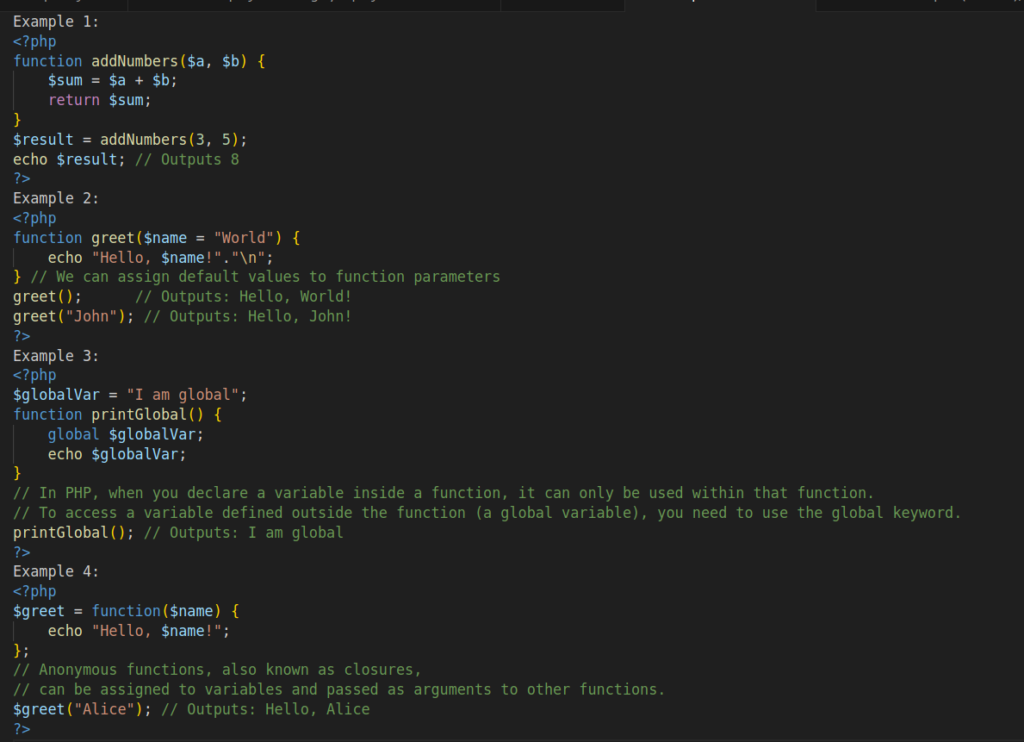
Arrays
We have already discussed the array concepts in the previous section on “A: Array Adventures“.
Strings
- Strings can be declared using single quotes (‘) or double quotes (“).
- String Concatenation is the process of combining two or more strings where you can use the `.` operator and `.=` is used for the assignment operator.
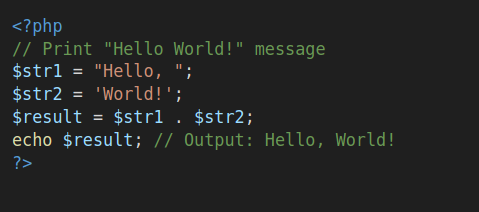
- You can find the length of a string using the `strlen()` function.
- You can pick out a specific letter from a word by using square brackets and indicating its position in the word.
- You can use the `substr()` function to extract a portion of a string.
- You can use a variety of useful functions in PHP for handling strings. Some examples include the `strtolower()`, `strtoupper()`, `strpos()`, `str_replace()`, and `trim()` functions make it easy to perform common string manipulations in PHP.
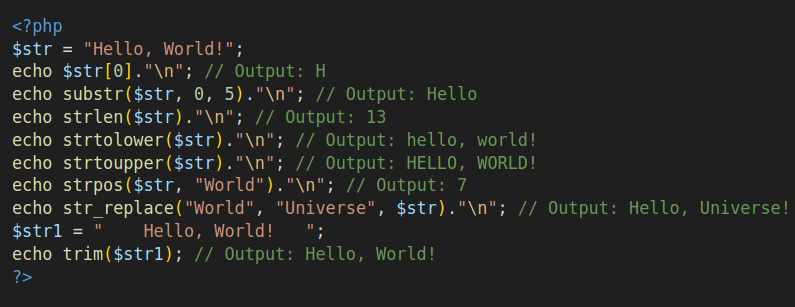